mirror of
https://github.com/pissnet/pissircd.git
synced 2025-07-18 17:12:25 +01:00
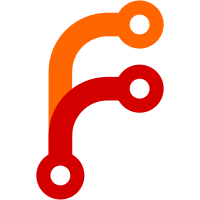
later fd_close() call. This also removes fd_map() since fd_open w/FDCLOSE_NONE now does that. * If you use fd_socket() or fd_accept(), then no change. When fd_close() is called we call close() on *NIX and closesocket() on Win. * If you use fd_fileopen(), then no change. When fd_close() is called we will call close() on both *NIX and Win. * If you used fd_open() and then fd_unmap() because you didn't want us to close the socket, then use fd_open() with FDCLOSE_NONE and just call fd_close() instead of fd_unmap(). We will not actually close the fd in fd_close() (FDCLOSE_NONE). * If you called fd_open() with other intentions then either specify a FDCLOSE_SOCKET / FDCLOSE_FILE as the last argument, or more likely: don't use fd_open() at all and use fd_socket() or fd_fileopen() instead. For reasons on this change, see previous patch. This way is more sane and makes it harder to make mistakes even beyond Windows-specific issues.
42 lines
1.2 KiB
C
42 lines
1.2 KiB
C
#ifndef FDLIST_H
|
|
#define FDLIST_H
|
|
|
|
/* $Id$ */
|
|
|
|
#define FD_DESC_SZ (100)
|
|
|
|
typedef void (*IOCallbackFunc)(int fd, int revents, void *data);
|
|
|
|
typedef enum FDCloseMethod { FDCLOSE_SOCKET=0, FDCLOSE_FILE=1, FDCLOSE_NONE=3 } FDCloseMethod;
|
|
|
|
typedef struct fd_entry {
|
|
int fd;
|
|
char desc[FD_DESC_SZ];
|
|
IOCallbackFunc read_callback;
|
|
IOCallbackFunc write_callback;
|
|
void *data;
|
|
time_t deadline;
|
|
unsigned char is_open;
|
|
FDCloseMethod close_method;
|
|
unsigned int backend_flags;
|
|
} FDEntry;
|
|
|
|
extern MODVAR FDEntry fd_table[MAXCONNECTIONS + 1];
|
|
|
|
extern int fd_open(int fd, const char *desc, FDCloseMethod close_method);
|
|
extern int fd_close(int fd);
|
|
extern void fd_unnotify(int fd);
|
|
extern int fd_socket(int family, int type, int protocol, const char *desc);
|
|
extern int fd_accept(int sockfd);
|
|
extern void fd_desc(int fd, const char *desc);
|
|
extern int fd_fileopen(const char *path, unsigned int flags);
|
|
|
|
#define FD_SELECT_READ 0x1
|
|
#define FD_SELECT_WRITE 0x2
|
|
|
|
extern void fd_setselect(int fd, int flags, IOCallbackFunc iocb, void *data);
|
|
extern void fd_select(time_t delay); /* backend-specific */
|
|
extern void fd_refresh(int fd); /* backend-specific */
|
|
extern void fd_fork(); /* backend-specific */
|
|
|
|
#endif
|