mirror of
https://codeberg.org/noisytoot/notnotdnethack.git
synced 2025-07-05 13:07:10 +01:00
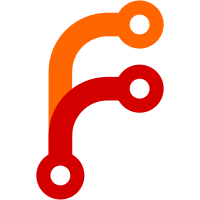
This creates the forge dungeon feature. This is a straight port, with no added functionality (and plenty of removed functionality) Currently mostly does nothing, pending integration w/ NPC smiths and creation of a smithing skill -Reforging to remove rust etc. in commented out (this is something smiths will be able to do but it needs to be integrated with whatever that system ends up being) -Blessing is commented out -The blacksmiths cookbook was not ported. Basic items can be made by NPC smiths, artifacts and magic items await a PC smithing skill -Can still break, dip into, and drink from forges --Dipping destroys non-metal items, and can be used to burn up straitjackets/striped shirts (in extchange for 6d6 damage!) --Dipping can ocasionally summon monsters and blow up forges --Dipping with a wielded hammer allows unpunishing --Drinking from is still an instadeath in most cases. --Breaking results in lava and is probably a very bad idea. Ports fire damage function improvements and lava damage (from 3.6? .7? vanilla?)
265 lines
4.5 KiB
C
265 lines
4.5 KiB
C
/* SCCS Id: @(#)sp_lev.h 3.4 1996/05/08 */
|
|
/* Copyright (c) 1989 by Jean-Christophe Collet */
|
|
/* NetHack may be freely redistributed. See license for details. */
|
|
|
|
#ifndef SP_LEV_H
|
|
#define SP_LEV_H
|
|
|
|
/* wall directions */
|
|
#define W_NORTH 1
|
|
#define W_SOUTH 2
|
|
#define W_EAST 4
|
|
#define W_WEST 8
|
|
#define W_ANY (W_NORTH|W_SOUTH|W_EAST|W_WEST)
|
|
|
|
/* MAP limits */
|
|
#define MAP_X_LIM 76
|
|
#define MAP_Y_LIM 21
|
|
|
|
/* Per level flags */
|
|
#define NOTELEPORT 0x00000001L
|
|
#define HARDFLOOR 0x00000002L
|
|
#define NOMMAP 0x00000004L
|
|
#define SHORTSIGHTED 0x00000008L
|
|
#define ARBOREAL 0x00000010L
|
|
#define LETHE 0x00000020L /* All water on level is Lethe-ized */
|
|
#define SLIME 0x00000040L
|
|
#define FUNGI 0x00000080L
|
|
#define DUN 0x00000100L
|
|
#define NECRO 0x00000200L
|
|
#define CAVE 0x00000400L
|
|
#define OUTSIDE 0x00000800L
|
|
|
|
/* special level types */
|
|
#define SP_LEV_ROOMS 1
|
|
#define SP_LEV_MAZE 2
|
|
|
|
/*
|
|
* Structures manipulated by the special levels loader & compiler
|
|
*/
|
|
|
|
typedef union str_or_len {
|
|
char *str;
|
|
int len;
|
|
} Str_or_Len;
|
|
|
|
typedef struct {
|
|
boolean init_present, padding;
|
|
char fg, bg;
|
|
boolean smoothed, joined;
|
|
xchar lit, walled;
|
|
} lev_init;
|
|
|
|
typedef struct {
|
|
xchar x, y, mask;
|
|
short arti_text; /* Index of the text string for this (artifact) door */
|
|
} door;
|
|
|
|
typedef struct {
|
|
xchar wall, pos, secret, mask;
|
|
// short arti_key; /* Index (ART_) of key for this door */
|
|
short arti_text; /* Index of the text string for this door */
|
|
} room_door;
|
|
|
|
typedef struct {
|
|
xchar x, y, chance, type;
|
|
} trap;
|
|
|
|
typedef struct {
|
|
Str_or_Len name, appear_as;
|
|
short id;
|
|
aligntyp align;
|
|
xchar x, y, chance, class, appear;
|
|
schar peaceful, asleep;
|
|
} monster;
|
|
|
|
typedef struct {
|
|
Str_or_Len name;
|
|
int corpsenm;
|
|
short id, spe;
|
|
xchar x, y, chance, class, containment;
|
|
schar curse_state;
|
|
} object;
|
|
|
|
typedef struct {
|
|
xchar x, y;
|
|
aligntyp align;
|
|
xchar shrine;
|
|
int god;
|
|
} altar;
|
|
|
|
typedef struct {
|
|
xchar x, y, dir, db_open;
|
|
} drawbridge;
|
|
|
|
typedef struct {
|
|
xchar x, y, dir;
|
|
} walk;
|
|
|
|
typedef struct {
|
|
xchar x1, y1, x2, y2;
|
|
} digpos;
|
|
|
|
typedef struct {
|
|
xchar x, y, up;
|
|
} lad;
|
|
|
|
typedef struct {
|
|
xchar x, y, up;
|
|
} stair;
|
|
|
|
typedef struct {
|
|
xchar x1, y1, x2, y2;
|
|
xchar rtype, rlit, rirreg;
|
|
} region;
|
|
|
|
/* values for rtype are defined in dungeon.h */
|
|
typedef struct {
|
|
struct { xchar x1, y1, x2, y2; } inarea;
|
|
struct { xchar x1, y1, x2, y2; } delarea;
|
|
boolean in_islev, del_islev;
|
|
xchar rtype, padding;
|
|
Str_or_Len rname;
|
|
} lev_region;
|
|
|
|
typedef struct {
|
|
xchar x, y;
|
|
int amount;
|
|
} gold;
|
|
|
|
typedef struct {
|
|
xchar x, y;
|
|
Str_or_Len engr;
|
|
xchar etype;
|
|
} engraving;
|
|
|
|
typedef struct {
|
|
xchar x, y;
|
|
} fountain;
|
|
|
|
typedef struct {
|
|
xchar x, y;
|
|
} forge;
|
|
|
|
typedef struct {
|
|
xchar x, y;
|
|
} sink;
|
|
|
|
typedef struct {
|
|
xchar x, y;
|
|
} pool;
|
|
|
|
typedef struct {
|
|
char halign, valign;
|
|
char xsize, ysize;
|
|
char **map;
|
|
char nrobjects;
|
|
char *robjects;
|
|
char nloc;
|
|
char *rloc_x;
|
|
char *rloc_y;
|
|
char nrmonst;
|
|
char *rmonst;
|
|
char nreg;
|
|
region **regions;
|
|
char nlreg;
|
|
lev_region **lregions;
|
|
char ndoor;
|
|
door **doors;
|
|
char ntrap;
|
|
trap **traps;
|
|
char nmonster;
|
|
monster **monsters;
|
|
char nobject;
|
|
object **objects;
|
|
char ndrawbridge;
|
|
drawbridge **drawbridges;
|
|
char nwalk;
|
|
walk **walks;
|
|
char ndig;
|
|
digpos **digs;
|
|
char npass;
|
|
digpos **passs;
|
|
char nlad;
|
|
lad **lads;
|
|
char nstair;
|
|
stair **stairs;
|
|
char naltar;
|
|
altar **altars;
|
|
char ngold;
|
|
gold **golds;
|
|
char nengraving;
|
|
engraving **engravings;
|
|
char nfountain;
|
|
fountain **fountains;
|
|
char nforge;
|
|
forge **forges;
|
|
} mazepart;
|
|
|
|
typedef struct {
|
|
long flags;
|
|
lev_init init_lev;
|
|
schar filling;
|
|
char numpart;
|
|
mazepart **parts;
|
|
} specialmaze;
|
|
|
|
typedef struct _room {
|
|
char *name;
|
|
char *parent;
|
|
xchar x, y, w, h;
|
|
xchar xalign, yalign;
|
|
xchar rtype, chance, rlit, filled;
|
|
char ndoor;
|
|
room_door **doors;
|
|
char ntrap;
|
|
trap **traps;
|
|
char nmonster;
|
|
monster **monsters;
|
|
char nobject;
|
|
object **objects;
|
|
char naltar;
|
|
altar **altars;
|
|
char nstair;
|
|
stair **stairs;
|
|
char ngold;
|
|
gold **golds;
|
|
char nengraving;
|
|
engraving **engravings;
|
|
char nfountain;
|
|
fountain **fountains;
|
|
char nforge;
|
|
forge **forges;
|
|
char nsink;
|
|
sink **sinks;
|
|
char npool;
|
|
pool **pools;
|
|
/* These three fields are only used when loading the level... */
|
|
int nsubroom;
|
|
struct _room *subrooms[MAX_SUBROOMS];
|
|
struct mkroom *mkr;
|
|
} room;
|
|
|
|
typedef struct {
|
|
struct {
|
|
xchar room;
|
|
xchar wall;
|
|
xchar door;
|
|
} src, dest;
|
|
} corridor;
|
|
|
|
/* used only by lev_comp */
|
|
typedef struct {
|
|
long flags;
|
|
lev_init init_lev;
|
|
char nrobjects;
|
|
char *robjects;
|
|
char nrmonst;
|
|
char *rmonst;
|
|
xchar nroom;
|
|
room **rooms;
|
|
xchar ncorr;
|
|
corridor **corrs;
|
|
} splev;
|
|
|
|
#endif /* SP_LEV_H */
|